はじめに
用語解説
CI/CDとは?
- コードの変更:開発者がコードを変更し、リポジトリにプッシュします。
- 自動ビルドとテスト (CI):CodeBuildなどのツールがコードをビルドし、自動テストを実行します。
- デプロイ (CD):CodeDeployがビルドされたコードをターゲット環境にデプロイします。
buildspecとは?
jestとは?
環境準備の手順
環境構成
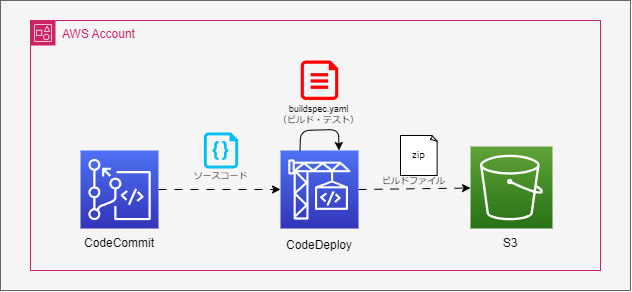
- CodeBuildでビルドを実行し、CodeCommitからソースを取得
- ビルド・テストを実施
- ビルドしたファイルをzip化しS3にアップロード
S3バケットの作成
アーティファクト(ビルドしたファイル)格納用のS3バケットの作成

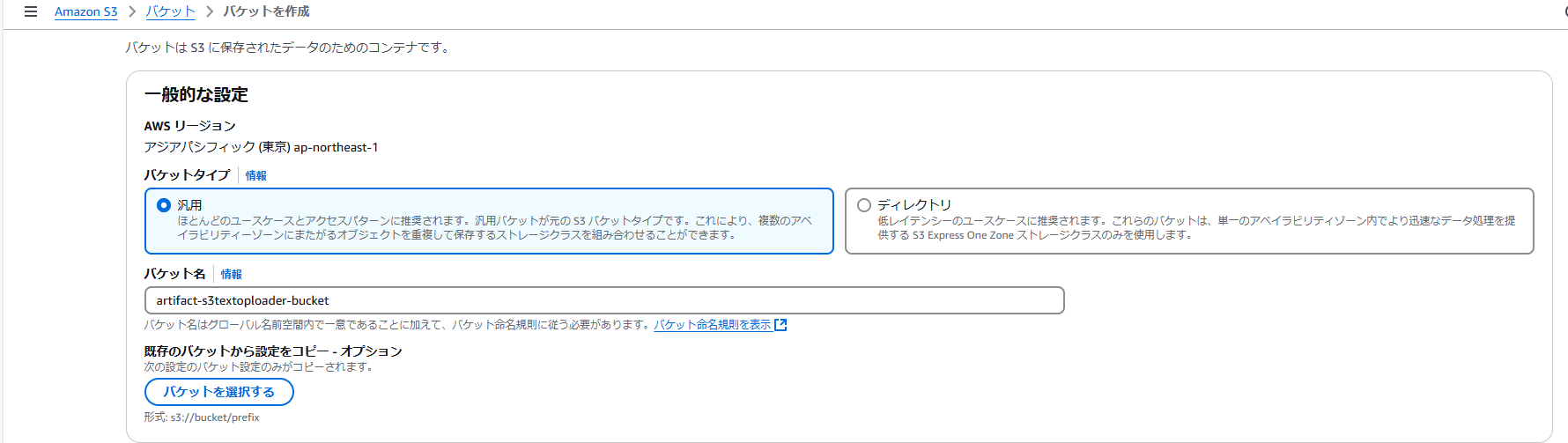
CodeCommitでリポジトリを作成
リポジトリの作成
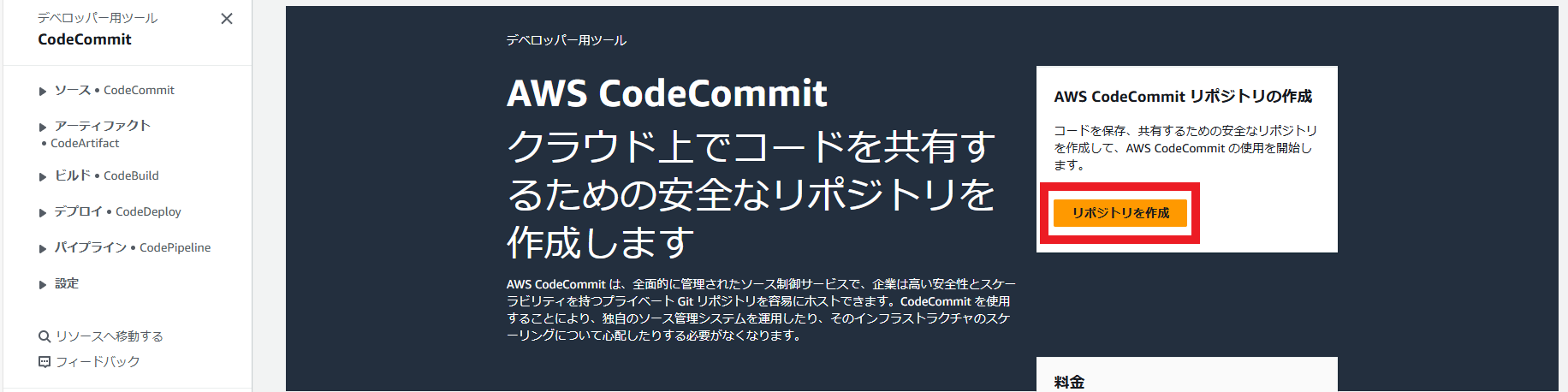
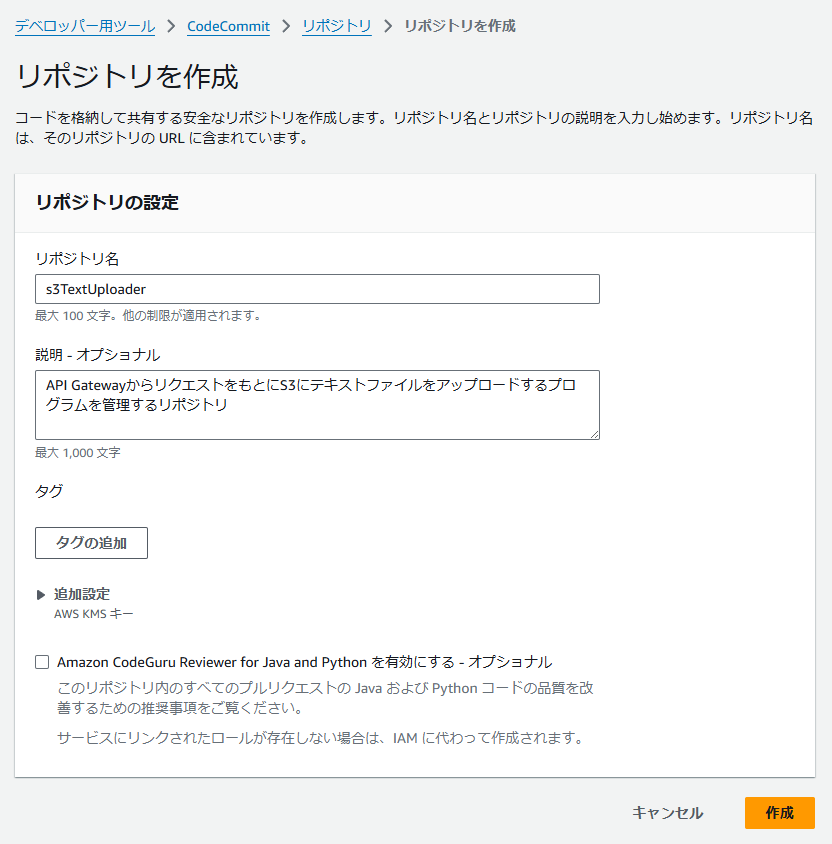
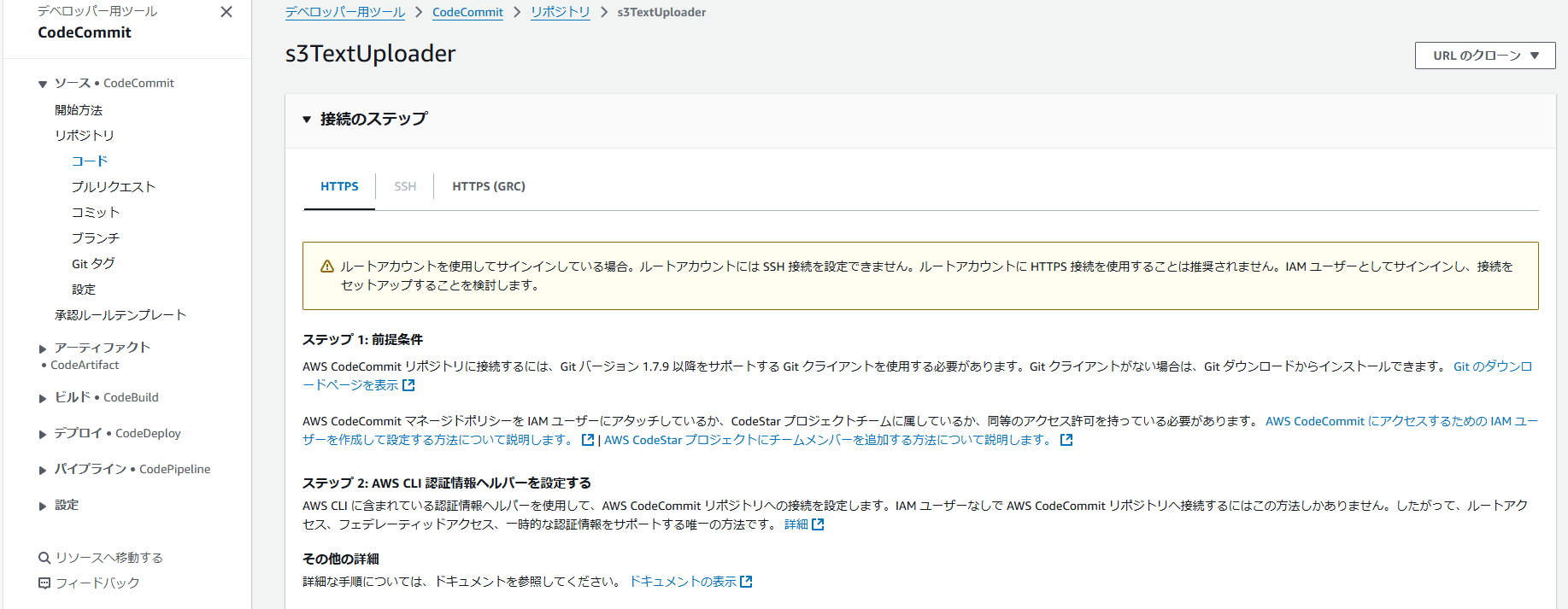
リポジトリの設定
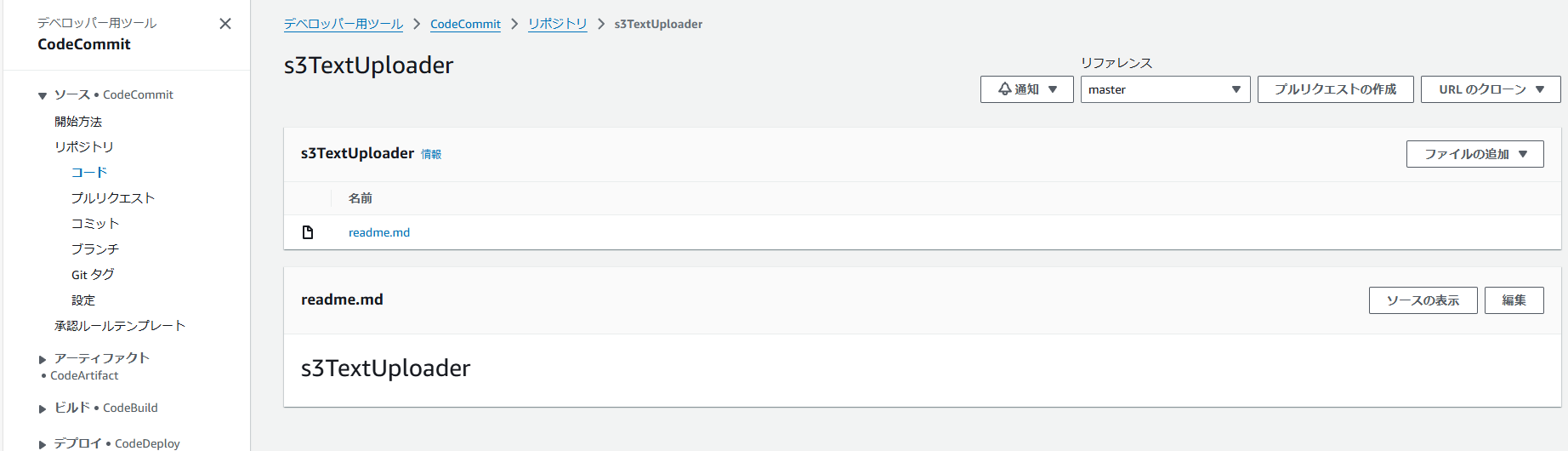
CodeBuildの設定
CodeBuildでビルドの設定します。
CodeBuildの画面を開き、プロジェクトを作成します。プロジェクトの作成を選択してください。
プロジェクトの作成画面が表示されます。
以下を参考に設定してください。
※今回は紹介のためmasterブランチを使ってます。実際のビルドの計画に合わせてブランチ名を修正してください。
ソース・テストコードの作成
開発環境のセットアップ
以下コマンドでソースとテストコードを取得できます。
.gitフォルダ以外をフォルダ・ファイルをCodeCommitのローカルリポジトリにコピーして、リモートリポジトリにプッシュしてください。
git clone https://github.com/TeTeTe-Jack/aws-handson-s3-text-uploader.git
/
├─ __test__
│ ├─ handler.spec.ts
│ └─ setEnv.ts
├─ coverage // カバレッジの出力で作成される テストをするまではかからフォルダ
│ └─ (カバレッジの出力ファイル)
├─ dist // ビルドの実行で作成される ビルドをするまではかからフォルダ
│ └─ (ビルドの成果物ファイル)
├─ node_modules
│ └─ (各外部モジュール)
├─ report // テストの実行で作成される テストをするまではかからフォルダ
│ └─ (テストの結果ファイル)
├─ src
│ └─ handler.ts
├─ .gitignore
├─ buildspec.yml
├─ jest.config.ts
├─ package-lock.json
├─ package.json
├─ readme.md
└─ tsconfig.json
開発用のフォルダを作成しnodeの初期設定します。
npm init -y
"scripts": {
"build": "tsc",
"test": "jest --coverage"
},
npm install --save-dev typescript @types/aws-lambda @aws-sdk/client-s3 @types/jest jest ts-jest ts-node jest-junit
npx tsc --init
修正ファイル:tsconfig.json
{
"compilerOptions": {
"target": "ES2022",
"module": "commonjs",
"outDir": "./dist/",
"forceConsistentCasingInFileNames": true,
"strict": true,
"skipLibCheck": true
},
"include": [
"src/*.ts"
],
"exclude": [
"node_modules"
]
}
npx jest --init
修正ファイル:jest.config.json
module.exports = {
reporters: [
'default',
[ 'jest-junit', {
outputDirectory: "report",
outputName: "report.xml",
} ]
],
transform: {
"^.+\\.tsx?$": "ts-jest",
},
moduleFileExtensions: ["ts", "tsx", "js"],
testEnvironment: "node",
globalSetup: "/__test__/setEnv.ts",
};
version: 0.2
phases:
install:
commands:
- echo "Install started on `TZ=-9 date '+%Y/%m/%d %H:%M:%S JST'`"
- echo "Install ended on `TZ=-9 date '+%Y/%m/%d %H:%M:%S JST'`"
pre_build:
commands:
- echo "Pre-Build started on `TZ=-9 date '+%Y/%m/%d %H:%M:%S JST'`"
- npm ci
- echo "Pre-Build ended on `TZ=-9 date '+%Y/%m/%d %H:%M:%S JST'`"
build:
commands:
- echo "Build started on `TZ=-9 date '+%Y/%m/%d %H:%M:%S JST'`"
- npm run build
- npm run test
- echo "Build ended on `TZ=-9 date '+%Y/%m/%d %H:%M:%S JST'`"
post_build:
commands:
- echo "Post-Build started on `TZ=-9 date '+%Y/%m/%d %H:%M:%S JST'`"
- echo "Post-Build ended on `TZ=-9 date '+%Y/%m/%d %H:%M:%S JST'`"
reports:
jest_reports:
files:
- report.xml
file-format: JUNITXML
base-directory: report
coverage_reports:
files:
- clover.xml
file-format: CLOVERXML
base-directory: coverage
artifacts:
files:
- ./**/*
base-directory: dist
# 外部モジュールの格納先
/node_modules
# ビルドしたファイルの格納先
/dist
# テストのレポートの格納先
/report
# コードカバレッジのレポートの格納先
/coverage
mkdir src
mkdir __test__
/
├─ __test__
│ └─ (テストコード)
├─ coverage // カバレッジの出力で作成される
│ └─ (カバレッジの出力ファイル)
├─ dist // ビルドの実行で作成される
│ └─ (ビルドの成果物ファイル)
├─ node_modules
│ └─ (各外部モジュール)
├─ report // テストの実行で作成される
│ └─ (テストの結果ファイル)
├─ src
│ └─ (ソースファイル)
├─ .gitignore
├─ buildspec.yml
├─ jest.config.ts
├─ package-lock.json
├─ package.json
├─ readme.md
└─ tsconfig.json
ソースとテストコード
import { S3Client, PutObjectCommand } from "@aws-sdk/client-s3";
import { APIGatewayProxyHandlerV2 } from "aws-lambda";
const s3Client = new S3Client({ region: process.env.AWS_REGION });
const BUCKET_NAME = process.env.BUCKET_NAME;
export const handler: APIGatewayProxyHandlerV2 = async (event) => {
try {
// Validate query parameters
const { queryStringParameters } = event;
if (!queryStringParameters) {
return {
statusCode: 400,
body: JSON.stringify({ message: "Missing query parameters" }),
};
}
const { filename, content } = queryStringParameters;
if (!filename || !content) {
return {
statusCode: 400,
body: JSON.stringify({ message: "Both 'filename' and 'content' parameters are required" }),
};
}
// Upload content to S3
const uploadParams = {
Bucket: BUCKET_NAME,
Key: filename,
Body: content,
ContentType: "text/plain",
};
await s3Client.send(new PutObjectCommand(uploadParams));
return {
statusCode: 200,
body: JSON.stringify({
message: "File uploaded successfully",
filename,
}),
};
} catch (error) {
console.error("Error uploading file to S3:", error);
return {
statusCode: 500,
body: JSON.stringify({
message: "Internal Server Error",
error: error instanceof Error ? error.message : "Unknown error",
}),
};
}
};
export default (): void => {
console.log("\nSetup test environment");
process.env.AWS_REGION = "ap-northeast-1";
process.env.BUCKET_NAME = "test-bucket";
console.log("process.env.AWS_REGION", process.env.AWS_REGION);
console.log("process.env.BUCKET_NAME", process.env.BUCKET_NAME);
};
import { handler } from "./../src/handler";
import { S3Client } from "@aws-sdk/client-s3";
import { Context, APIGatewayProxyResult } from "aws-lambda";
jest.mock("@aws-sdk/client-s3", () => {
const mockS3Client = {
send: jest.fn(),
};
return {
S3Client: jest.fn(() => mockS3Client),
PutObjectCommand: jest.fn((params) => params),
};
});
describe("Lambda Function - Upload to S3", () => {
const mockS3Client = new S3Client({});
const mockEnv = process.env;
beforeEach(() => {
jest.clearAllMocks();
process.env = { ...mockEnv, BUCKET_NAME: "test-bucket" };
});
afterAll(() => {
process.env = mockEnv;
});
const mockContext: Context = {
awsRequestId: "test-request-id",
callbackWaitsForEmptyEventLoop: false,
functionName: "test-function",
functionVersion: "$LATEST",
invokedFunctionArn: "arn:aws:lambda:us-east-1:123456789012:function:test-function",
logGroupName: "/aws/lambda/test-function",
logStreamName: "test-log-stream",
memoryLimitInMB: "128",
getRemainingTimeInMillis: () => 3000,
done: () => {},
fail: () => {},
succeed: () => {},
} as Context;
it("should return 400 if query parameters are missing", async () => {
const event = { queryStringParameters: null } as any;
const result = (await handler(event, mockContext, () => {})) as APIGatewayProxyResult;
expect(result.statusCode).toBe(400);
expect(result.body).toContain("Missing query parameters");
});
it("should return 400 if 'filename' or 'content' is missing", async () => {
const event = {
queryStringParameters: { filename: "test.txt" },
} as any;
const result = (await handler(event, mockContext, () => {})) as APIGatewayProxyResult;
expect(result.statusCode).toBe(400);
expect(result.body).toContain("Both 'filename' and 'content' parameters are required");
});
it("should upload file to S3 and return 200", async () => {
const event = {
queryStringParameters: {
filename: "test.txt",
content: "Hello World",
},
} as any;
(mockS3Client.send as jest.Mock).mockImplementation((command) => {
return Promise.resolve(command.input);
});
const result = (await handler(event, mockContext, () => {})) as APIGatewayProxyResult;
expect(result.statusCode).toBe(200);
expect(result.body).toContain("File uploaded successfully");
expect(mockS3Client.send).toHaveBeenCalledWith(
expect.objectContaining({
Bucket: "test-bucket",
Key: "test.txt",
Body: "Hello World",
})
);
});
it("should return 500 if S3 upload fails", async () => {
const event = {
queryStringParameters: {
filename: "test.txt",
content: "Hello World",
},
} as any;
(mockS3Client.send as jest.Mock).mockRejectedValueOnce(new Error("S3 upload error"));
const result = (await handler(event, mockContext, () => {})) as APIGatewayProxyResult;
expect(result.statusCode).toBe(500);
expect(result.body).toContain("Internal Server Error");
expect(result.body).toContain("S3 upload error");
});
it("should return 500 if unkwon error", async () => {
const event = {
queryStringParameters: {
filename: "test.txt",
content: "Hello World",
},
} as any;
(mockS3Client.send as jest.Mock).mockRejectedValueOnce("test");
const result = (await handler(event, mockContext, () => {})) as APIGatewayProxyResult;
expect(result.statusCode).toBe(500);
expect(result.body).toContain("Internal Server Error");
expect(result.body).toContain("Unknown error");
});
});
CodeCommitにpush
git add *
git commit -m "add setting files and source files"
git push
動作確認
CodeBuildの画面を開きます。
左メニューのビルドプロジェクトを選択します。
作成したプロジェクトを選択します。
ビルドを開始を選択します
ビルドを開始するとビルドログが表示されます。エラーなどが発生した場合はこちらのログを確認してください。
フェーズ詳細タブを選択するとビルドがどこまで進んでいるかわかります。COMPLETEが成功となっていればビルド完了です。
レポートタブでテスト結果を確認することができます。今回はテストとテストのコードカバレッジをレポートに出力しています。
テストの結果から確認していきます。
今回の結果はテストが100%クリアとなってます。各テストケースで出力メッセージを確認することができるので失敗時に参考にしてみてください。
続いてコードカバレッジを確認していきます。
ラインカバレッジとブランチカバレッジがともに100%となりました。
最後にビルド成果物(アーティファクト)の確認をします。S3の画面を開き汎用バケットを選択してください。
S3バケット一覧が表示されるので、アーティファクト格納用で作成したS3バケットを選択します。
artifact.zipが格納されています。こちらのzipファイルを使ってLambda関数のコードの更新が可能です。
zipファイルをダウンロードして、解凍してみると、JavaScriptに変換されたファイルが格納されていました。
まとめ
この記事では、AWSを使ったCI/CDのはじめの一歩として、CodeBuildを使ってビルド・テストをする方法を解説しました。
CodeBuildを使ったビルドやテストについて流れをつかめたのではないかと思います。
今回ビルド・テストの方法を応用することで、CodeCommitのイベントをトリガーにして、ビルドやデプロイをすることも可能です。
より詳しく知りたい方は以下の書籍がおすすめです。